mirror of
https://github.com/micku7zu/vanilla-tilt.js
synced 2024-06-22 07:16:27 +02:00
Rename vanillaTilt.js to vanilla-tilt.js
This commit is contained in:
parent
4ed021d6b0
commit
08acafa790
14
README.md
14
README.md
|
@ -1,11 +1,11 @@
|
|||
# vanillaTilt.js. Tilt.js without jQuery.
|
||||
# vanilla-tilt.js
|
||||
Rewritten from [Tilt.js](https://github.com/gijsroge/tilt.js).
|
||||
**All copyright goes to [https://github.com/gijsroge/tilt.js](https://github.com/gijsroge/tilt.js)**
|
||||
|
||||
A tiny requestAnimationFrame powered 60+fps lightweight parallax tilt effect without any dependencies.
|
||||
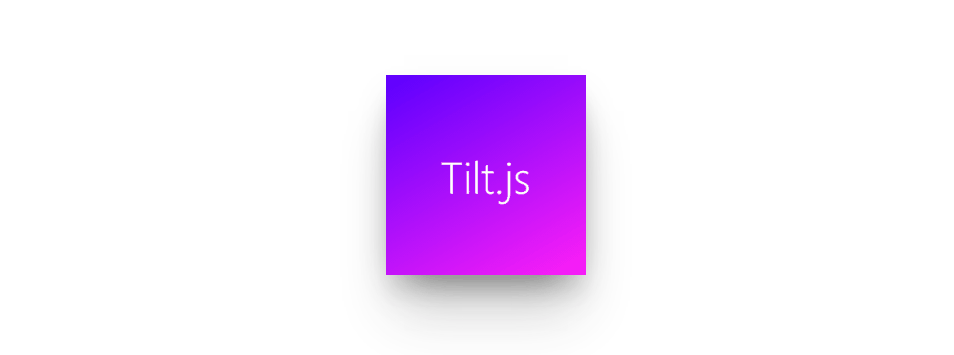
|
||||
|
||||
####Take a look at the **[landing page](https://micku7zu.github.io/vanillaTilt.js/index.html)** for demo's.
|
||||
####Take a look at the **[landing page](https://micku7zu.github.io/vanilla-tilt.js/index.html)** for demo's.
|
||||
|
||||
### Usage
|
||||
|
||||
|
@ -13,7 +13,7 @@ A tiny requestAnimationFrame powered 60+fps lightweight parallax tilt effect wit
|
|||
<!DOCTYPE html>
|
||||
<body>
|
||||
<div data-tilt></div> <!-- Tilt element -->
|
||||
<script src="vanillaTilt.js"></script> <!-- Load vanillaTilt.js library -->
|
||||
<script src="vanilla-tilt.js"></script> <!-- Load vanilla-tilt.js library -->
|
||||
</body>
|
||||
```
|
||||
|
||||
|
@ -54,9 +54,9 @@ element.vanillaTilt.reset();
|
|||
### Install
|
||||
You can copy and include any of the following file:
|
||||
|
||||
* dest/vanillaTilt.js ~ 6kb
|
||||
* dest/vanillaTilt.min.js ~ 3.5kb
|
||||
* dest/vanillaTilt.babel.js ~ 8.5kb
|
||||
* dest/vanillaTilt.babel.min.js ~ 4.3kb
|
||||
* dest/vanilla-tilt.js ~ 6kb
|
||||
* dest/vanilla-tilt.min.js ~ 3.5kb
|
||||
* dest/vanilla-tilt.babel.js ~ 8.5kb
|
||||
* dest/vanilla-tilt.babel.min.js ~ 4.3kb
|
||||
|
||||
in your webiste.
|
|
@ -3,7 +3,7 @@
|
|||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<title>VanillaTilt.js</title>
|
||||
<title>vanilla-tilt.js</title>
|
||||
<link href="https://fonts.googleapis.com/css?family=Source+Sans+Pro:300,400,400i,600,700" rel="stylesheet">
|
||||
<link href="https://fonts.googleapis.com/css?family=Fira+Sans+Extra+Condensed" rel="stylesheet">
|
||||
<style>
|
||||
|
@ -88,7 +88,7 @@
|
|||
<body>
|
||||
|
||||
<header>
|
||||
<h1><span class="js-tilt">vanillaTilt</span><span class="js-tilt">.js</span></h1>
|
||||
<h1><span class="js-tilt">vanilla-tilt</span><span class="js-tilt">.js</span></h1>
|
||||
<p>
|
||||
A tiny requestAnimationFrame powered 60+fps lightweight parallax tilt effect rewritten without any dependencies.
|
||||
</p>
|
||||
|
@ -107,7 +107,7 @@
|
|||
|
||||
<br><br><br><br><br><br><br><br><br><br><br><br><br><br><br><br>
|
||||
</header>
|
||||
<script src="../dest/vanillaTilt.js"></script>
|
||||
<script src="../src/vanilla-tilt.js"></script>
|
||||
<script>
|
||||
VanillaTilt.init(document.querySelectorAll(".js-tilt"), {
|
||||
speed: 400,
|
||||
|
|
|
@ -1,217 +0,0 @@
|
|||
/**
|
||||
* Created by micku7zu on 1/27/2017.
|
||||
* Original idea: http://gijsroge.github.io/tilt.js/
|
||||
* MIT License.
|
||||
* Version 1.0.0
|
||||
*/
|
||||
"use strict";
|
||||
|
||||
var _createClass = function () { function defineProperties(target, props) { for (var i = 0; i < props.length; i++) { var descriptor = props[i]; descriptor.enumerable = descriptor.enumerable || false; descriptor.configurable = true; if ("value" in descriptor) descriptor.writable = true; Object.defineProperty(target, descriptor.key, descriptor); } } return function (Constructor, protoProps, staticProps) { if (protoProps) defineProperties(Constructor.prototype, protoProps); if (staticProps) defineProperties(Constructor, staticProps); return Constructor; }; }();
|
||||
|
||||
function _classCallCheck(instance, Constructor) { if (!(instance instanceof Constructor)) { throw new TypeError("Cannot call a class as a function"); } }
|
||||
|
||||
(function (undefined) {
|
||||
var VanillaTilt = function () {
|
||||
function VanillaTilt(element) {
|
||||
var settings = arguments.length > 1 && arguments[1] !== undefined ? arguments[1] : {};
|
||||
|
||||
_classCallCheck(this, VanillaTilt);
|
||||
|
||||
if (!(element instanceof Node)) {
|
||||
throw "Can't initialize VanillaTilt because " + element + " is not a Node.";
|
||||
}
|
||||
|
||||
this.width = null;
|
||||
this.height = null;
|
||||
this.left = null;
|
||||
this.top = null;
|
||||
this.transitionTimeout = null;
|
||||
this.updateCall = null;
|
||||
|
||||
this.updateBind = this.update.bind(this);
|
||||
|
||||
this.element = element;
|
||||
this.settings = this.extendSettings(settings);
|
||||
|
||||
this.addEventListeners();
|
||||
}
|
||||
|
||||
_createClass(VanillaTilt, [{
|
||||
key: "addEventListeners",
|
||||
value: function addEventListeners() {
|
||||
this.onMouseEnterBind = this.onMouseEnter.bind(this);
|
||||
this.onMouseMoveBind = this.onMouseMove.bind(this);
|
||||
this.onMouseLeaveBind = this.onMouseLeave.bind(this);
|
||||
|
||||
this.element.addEventListener("mouseenter", this.onMouseEnterBind);
|
||||
this.element.addEventListener("mousemove", this.onMouseMoveBind);
|
||||
this.element.addEventListener("mouseleave", this.onMouseLeaveBind);
|
||||
}
|
||||
}, {
|
||||
key: "removeEventListeners",
|
||||
value: function removeEventListeners() {
|
||||
this.element.removeEventListener("mouseenter", this.onMouseEnterBind);
|
||||
this.element.removeEventListener("mousemove", this.onMouseMoveBind);
|
||||
this.element.removeEventListener("mouseleave", this.onMouseLeaveBind);
|
||||
}
|
||||
}, {
|
||||
key: "destroy",
|
||||
value: function destroy() {
|
||||
this.removeEventListeners();
|
||||
this.element.vanillaTilt = null;
|
||||
this.element = null;
|
||||
}
|
||||
}, {
|
||||
key: "onMouseEnter",
|
||||
value: function onMouseEnter(event) {
|
||||
this.width = this.element.offsetWidth;
|
||||
this.height = this.element.offsetHeight;
|
||||
this.left = this.element.offsetLeft;
|
||||
this.top = this.element.offsetTop;
|
||||
|
||||
this.element.style.willChange = "transform";
|
||||
this.setTransition();
|
||||
}
|
||||
}, {
|
||||
key: "onMouseMove",
|
||||
value: function onMouseMove(event) {
|
||||
if (this.updateCall !== null) {
|
||||
cancelAnimationFrame(this.updateCall);
|
||||
}
|
||||
|
||||
this.event = event;
|
||||
this.updateCall = requestAnimationFrame(this.updateBind);
|
||||
}
|
||||
}, {
|
||||
key: "onMouseLeave",
|
||||
value: function onMouseLeave(event) {
|
||||
this.setTransition();
|
||||
|
||||
if (this.settings.reset) {
|
||||
this.reset();
|
||||
}
|
||||
}
|
||||
}, {
|
||||
key: "reset",
|
||||
value: function reset() {
|
||||
var _this = this;
|
||||
|
||||
requestAnimationFrame(function () {
|
||||
_this.event = {
|
||||
pageX: _this.left + _this.width / 2,
|
||||
pageY: _this.top + _this.height / 2
|
||||
};
|
||||
|
||||
_this.element.style.transform = "perspective(" + _this.settings.perspective + "px) " + "rotateX(0deg) " + "rotateY(0deg) " + "scale3d(1, 1, 1)";
|
||||
});
|
||||
}
|
||||
}, {
|
||||
key: "getValues",
|
||||
value: function getValues() {
|
||||
var x = (this.event.pageX - this.left) / this.width;
|
||||
var y = (this.event.pageY - this.top) / this.height;
|
||||
|
||||
x = Math.min(Math.max(x, 0), 1);
|
||||
y = Math.min(Math.max(y, 0), 1);
|
||||
|
||||
var tiltX = (this.settings.max / 2 - x * this.settings.max).toFixed(2);
|
||||
var tiltY = (y * this.settings.max - this.settings.max / 2).toFixed(2);
|
||||
|
||||
return {
|
||||
tiltX: tiltX,
|
||||
tiltY: tiltY,
|
||||
percentageX: x * 100,
|
||||
percentageY: y * 100
|
||||
};
|
||||
}
|
||||
}, {
|
||||
key: "update",
|
||||
value: function update() {
|
||||
var values = this.getValues();
|
||||
|
||||
this.element.style.transform = "perspective(" + this.settings.perspective + "px) " + "rotateX(" + (this.settings.axis === "x" ? 0 : values.tiltY) + "deg) " + "rotateY(" + (this.settings.axis === "y" ? 0 : values.tiltX) + "deg) " + "scale3d(" + this.settings.scale + ", " + this.settings.scale + ", " + this.settings.scale + ")";
|
||||
|
||||
this.element.dispatchEvent(new CustomEvent("tiltChange", {
|
||||
"detail": values
|
||||
}));
|
||||
|
||||
this.updateCall = null;
|
||||
}
|
||||
}, {
|
||||
key: "setTransition",
|
||||
value: function setTransition() {
|
||||
var _this2 = this;
|
||||
|
||||
clearTimeout(this.transitionTimeout);
|
||||
this.element.style.transition = this.settings.speed + "ms " + this.settings.easing;
|
||||
this.transitionTimeout = setTimeout(function () {
|
||||
return _this2.element.style.transition = "";
|
||||
}, this.settings.speed);
|
||||
}
|
||||
}, {
|
||||
key: "extendSettings",
|
||||
value: function extendSettings(settings) {
|
||||
var defaultSettings = {
|
||||
max: 20,
|
||||
perspective: 1000,
|
||||
easing: "cubic-bezier(.03,.98,.52,.99)",
|
||||
scale: "1",
|
||||
speed: "300",
|
||||
transition: true,
|
||||
axis: null,
|
||||
reset: true
|
||||
};
|
||||
|
||||
var newSettings = {};
|
||||
|
||||
for (var property in defaultSettings) {
|
||||
if (property in settings) {
|
||||
newSettings[property] = settings[property];
|
||||
} else if (this.element.hasAttribute("data-tilt-" + property)) {
|
||||
var attribute = this.element.getAttribute("data-tilt-" + property);
|
||||
try {
|
||||
newSettings[property] = JSON.parse(attribute);
|
||||
} catch (e) {
|
||||
newSettings[property] = attribute;
|
||||
}
|
||||
} else {
|
||||
newSettings[property] = defaultSettings[property];
|
||||
}
|
||||
}
|
||||
|
||||
return newSettings;
|
||||
}
|
||||
}], [{
|
||||
key: "init",
|
||||
value: function init(elements, settings) {
|
||||
if (elements instanceof Node) {
|
||||
elements = [elements];
|
||||
}
|
||||
|
||||
if (elements instanceof NodeList) {
|
||||
elements = [].slice.call(elements);
|
||||
}
|
||||
|
||||
if (!(elements instanceof Array)) {
|
||||
return;
|
||||
}
|
||||
|
||||
elements.forEach(function (element) {
|
||||
element.vanillaTilt = new VanillaTilt(element, settings);
|
||||
});
|
||||
}
|
||||
}]);
|
||||
|
||||
return VanillaTilt;
|
||||
}();
|
||||
|
||||
/* expose the class to window */
|
||||
|
||||
|
||||
window.VanillaTilt = VanillaTilt;
|
||||
|
||||
/**
|
||||
* Auto load
|
||||
*/
|
||||
VanillaTilt.init(document.querySelectorAll("[data-tilt]"));
|
||||
})();
|
7
dest/vanillaTilt.babel.min.js
vendored
7
dest/vanillaTilt.babel.min.js
vendored
|
@ -1,7 +0,0 @@
|
|||
/**
|
||||
* Created by micku7zu on 1/27/2017.
|
||||
* Original idea: http://gijsroge.github.io/tilt.js/
|
||||
* MIT License.
|
||||
* Version 1.0.0
|
||||
*/
|
||||
"use strict";var _createClass=function(){function a(b,c){for(var f,d=0;d<c.length;d++)f=c[d],f.enumerable=f.enumerable||!1,f.configurable=!0,"value"in f&&(f.writable=!0),Object.defineProperty(b,f.key,f)}return function(b,c,d){return c&&a(b.prototype,c),d&&a(b,d),b}}();function _classCallCheck(a,b){if(!(a instanceof b))throw new TypeError("Cannot call a class as a function")}(function(){var b=function(){function c(d){var f=1<arguments.length&&void 0!==arguments[1]?arguments[1]:{};if(_classCallCheck(this,c),!(d instanceof Node))throw"Can't initialize VanillaTilt because "+d+" is not a Node.";this.width=null,this.height=null,this.left=null,this.top=null,this.transitionTimeout=null,this.updateCall=null,this.updateBind=this.update.bind(this),this.element=d,this.settings=this.extendSettings(f),this.addEventListeners()}return _createClass(c,[{key:"addEventListeners",value:function addEventListeners(){this.onMouseEnterBind=this.onMouseEnter.bind(this),this.onMouseMoveBind=this.onMouseMove.bind(this),this.onMouseLeaveBind=this.onMouseLeave.bind(this),this.element.addEventListener("mouseenter",this.onMouseEnterBind),this.element.addEventListener("mousemove",this.onMouseMoveBind),this.element.addEventListener("mouseleave",this.onMouseLeaveBind)}},{key:"removeEventListeners",value:function removeEventListeners(){this.element.removeEventListener("mouseenter",this.onMouseEnterBind),this.element.removeEventListener("mousemove",this.onMouseMoveBind),this.element.removeEventListener("mouseleave",this.onMouseLeaveBind)}},{key:"destroy",value:function destroy(){this.removeEventListeners(),this.element.vanillaTilt=null,this.element=null}},{key:"onMouseEnter",value:function onMouseEnter(){this.width=this.element.offsetWidth,this.height=this.element.offsetHeight,this.left=this.element.offsetLeft,this.top=this.element.offsetTop,this.element.style.willChange="transform",this.setTransition()}},{key:"onMouseMove",value:function onMouseMove(d){null!==this.updateCall&&cancelAnimationFrame(this.updateCall),this.event=d,this.updateCall=requestAnimationFrame(this.updateBind)}},{key:"onMouseLeave",value:function onMouseLeave(){this.setTransition(),this.settings.reset&&this.reset()}},{key:"reset",value:function reset(){var d=this;requestAnimationFrame(function(){d.event={pageX:d.left+d.width/2,pageY:d.top+d.height/2},d.element.style.transform="perspective("+d.settings.perspective+"px) rotateX(0deg) rotateY(0deg) scale3d(1, 1, 1)"})}},{key:"getValues",value:function getValues(){var d=(this.event.pageX-this.left)/this.width,f=(this.event.pageY-this.top)/this.height;d=Math.min(Math.max(d,0),1),f=Math.min(Math.max(f,0),1);var g=(this.settings.max/2-d*this.settings.max).toFixed(2),h=(f*this.settings.max-this.settings.max/2).toFixed(2);return{tiltX:g,tiltY:h,percentageX:100*d,percentageY:100*f}}},{key:"update",value:function update(){var d=this.getValues();this.element.style.transform="perspective("+this.settings.perspective+"px) rotateX("+("x"===this.settings.axis?0:d.tiltY)+"deg) rotateY("+("y"===this.settings.axis?0:d.tiltX)+"deg) scale3d("+this.settings.scale+", "+this.settings.scale+", "+this.settings.scale+")",this.element.dispatchEvent(new CustomEvent("tiltChange",{detail:d})),this.updateCall=null}},{key:"setTransition",value:function setTransition(){var d=this;clearTimeout(this.transitionTimeout),this.element.style.transition=this.settings.speed+"ms "+this.settings.easing,this.transitionTimeout=setTimeout(function(){return d.element.style.transition=""},this.settings.speed)}},{key:"extendSettings",value:function extendSettings(d){var g={max:20,perspective:1e3,easing:"cubic-bezier(.03,.98,.52,.99)",scale:"1",speed:"300",transition:!0,axis:null,reset:!0},h={};for(var f in g)if(f in d)h[f]=d[f];else if(this.element.hasAttribute("data-tilt-"+f)){var j=this.element.getAttribute("data-tilt-"+f);try{h[f]=JSON.parse(j)}catch(k){h[f]=j}}else h[f]=g[f];return h}}],[{key:"init",value:function init(d,f){d instanceof Node&&(d=[d]),d instanceof NodeList&&(d=[].slice.call(d)),d instanceof Array&&d.forEach(function(g){g.vanillaTilt=new c(g,f)})}}]),c}();window.VanillaTilt=b,b.init(document.querySelectorAll("[data-tilt]"))})();
|
|
@ -1,193 +0,0 @@
|
|||
/**
|
||||
* Created by micku7zu on 1/27/2017.
|
||||
* Original idea: http://gijsroge.github.io/tilt.js/
|
||||
* MIT License.
|
||||
* Version 1.0.0
|
||||
*/
|
||||
"use strict";
|
||||
(function (undefined) {
|
||||
|
||||
class VanillaTilt {
|
||||
constructor(element, settings = {}) {
|
||||
if (!(element instanceof Node)) {
|
||||
throw("Can't initialize VanillaTilt because " + element + " is not a Node.");
|
||||
}
|
||||
|
||||
this.width = null;
|
||||
this.height = null;
|
||||
this.left = null;
|
||||
this.top = null;
|
||||
this.transitionTimeout = null;
|
||||
this.updateCall = null;
|
||||
|
||||
this.updateBind = this.update.bind(this);
|
||||
|
||||
this.element = element;
|
||||
this.settings = this.extendSettings(settings);
|
||||
|
||||
this.addEventListeners();
|
||||
}
|
||||
|
||||
addEventListeners() {
|
||||
this.onMouseEnterBind = this.onMouseEnter.bind(this);
|
||||
this.onMouseMoveBind = this.onMouseMove.bind(this);
|
||||
this.onMouseLeaveBind = this.onMouseLeave.bind(this);
|
||||
|
||||
this.element.addEventListener("mouseenter", this.onMouseEnterBind);
|
||||
this.element.addEventListener("mousemove", this.onMouseMoveBind);
|
||||
this.element.addEventListener("mouseleave", this.onMouseLeaveBind);
|
||||
}
|
||||
|
||||
removeEventListeners() {
|
||||
this.element.removeEventListener("mouseenter", this.onMouseEnterBind);
|
||||
this.element.removeEventListener("mousemove", this.onMouseMoveBind);
|
||||
this.element.removeEventListener("mouseleave", this.onMouseLeaveBind);
|
||||
}
|
||||
|
||||
destroy() {
|
||||
this.removeEventListeners();
|
||||
this.element.vanillaTilt = null;
|
||||
this.element = null;
|
||||
}
|
||||
|
||||
onMouseEnter(event) {
|
||||
this.width = this.element.offsetWidth;
|
||||
this.height = this.element.offsetHeight;
|
||||
this.left = this.element.offsetLeft;
|
||||
this.top = this.element.offsetTop;
|
||||
|
||||
this.element.style.willChange = "transform";
|
||||
this.setTransition();
|
||||
}
|
||||
|
||||
onMouseMove(event) {
|
||||
if (this.updateCall !== null) {
|
||||
cancelAnimationFrame(this.updateCall);
|
||||
}
|
||||
|
||||
this.event = event;
|
||||
this.updateCall = requestAnimationFrame(this.updateBind);
|
||||
}
|
||||
|
||||
onMouseLeave(event) {
|
||||
this.setTransition();
|
||||
|
||||
if (this.settings.reset) {
|
||||
this.reset();
|
||||
}
|
||||
}
|
||||
|
||||
reset() {
|
||||
requestAnimationFrame(() => {
|
||||
this.event = {
|
||||
pageX: this.left + this.width / 2,
|
||||
pageY: this.top + this.height / 2
|
||||
};
|
||||
|
||||
this.element.style.transform = "perspective(" + this.settings.perspective + "px) " +
|
||||
"rotateX(0deg) " +
|
||||
"rotateY(0deg) " +
|
||||
"scale3d(1, 1, 1)";
|
||||
});
|
||||
}
|
||||
|
||||
getValues() {
|
||||
let x = (this.event.pageX - this.left) / this.width;
|
||||
let y = (this.event.pageY - this.top) / this.height;
|
||||
|
||||
x = Math.min(Math.max(x, 0), 1);
|
||||
y = Math.min(Math.max(y, 0), 1);
|
||||
|
||||
let tiltX = (this.settings.max / 2 - x * this.settings.max).toFixed(2);
|
||||
let tiltY = (y * this.settings.max - this.settings.max / 2).toFixed(2);
|
||||
|
||||
return {
|
||||
tiltX: tiltX,
|
||||
tiltY: tiltY,
|
||||
percentageX: x * 100,
|
||||
percentageY: y * 100
|
||||
};
|
||||
}
|
||||
|
||||
update() {
|
||||
let values = this.getValues();
|
||||
|
||||
this.element.style.transform = "perspective(" + this.settings.perspective + "px) " +
|
||||
"rotateX(" + (this.settings.axis === "x" ? 0 : values.tiltY) + "deg) " +
|
||||
"rotateY(" + (this.settings.axis === "y" ? 0 : values.tiltX) + "deg) " +
|
||||
"scale3d(" + this.settings.scale + ", " + this.settings.scale + ", " + this.settings.scale + ")";
|
||||
|
||||
|
||||
this.element.dispatchEvent(new CustomEvent("tiltChange", {
|
||||
"detail": values
|
||||
}));
|
||||
|
||||
this.updateCall = null;
|
||||
}
|
||||
|
||||
setTransition() {
|
||||
clearTimeout(this.transitionTimeout);
|
||||
this.element.style.transition = this.settings.speed + "ms " + this.settings.easing;
|
||||
this.transitionTimeout = setTimeout(() => this.element.style.transition = "", this.settings.speed);
|
||||
}
|
||||
|
||||
extendSettings(settings) {
|
||||
let defaultSettings = {
|
||||
max: 20,
|
||||
perspective: 1000,
|
||||
easing: "cubic-bezier(.03,.98,.52,.99)",
|
||||
scale: "1",
|
||||
speed: "300",
|
||||
transition: true,
|
||||
axis: null,
|
||||
reset: true
|
||||
};
|
||||
|
||||
let newSettings = {};
|
||||
|
||||
for (var property in defaultSettings) {
|
||||
if (property in settings) {
|
||||
newSettings[property] = settings[property];
|
||||
} else if (this.element.hasAttribute("data-tilt-" + property)) {
|
||||
let attribute = this.element.getAttribute("data-tilt-" + property);
|
||||
try {
|
||||
newSettings[property] = JSON.parse(attribute);
|
||||
} catch (e) {
|
||||
newSettings[property] = attribute;
|
||||
}
|
||||
|
||||
} else {
|
||||
newSettings[property] = defaultSettings[property];
|
||||
}
|
||||
}
|
||||
|
||||
return newSettings;
|
||||
}
|
||||
|
||||
static init(elements, settings) {
|
||||
if (elements instanceof Node) {
|
||||
elements = [elements];
|
||||
}
|
||||
|
||||
if (elements instanceof NodeList) {
|
||||
elements = [].slice.call(elements);
|
||||
}
|
||||
|
||||
if (!(elements instanceof Array)) {
|
||||
return;
|
||||
}
|
||||
|
||||
elements.forEach((element) => {
|
||||
element.vanillaTilt = new VanillaTilt(element, settings);
|
||||
});
|
||||
}
|
||||
}
|
||||
|
||||
/* expose the class to window */
|
||||
window.VanillaTilt = VanillaTilt;
|
||||
|
||||
/**
|
||||
* Auto load
|
||||
*/
|
||||
VanillaTilt.init(document.querySelectorAll("[data-tilt]"));
|
||||
})();
|
7
dest/vanillaTilt.min.js
vendored
7
dest/vanillaTilt.min.js
vendored
|
@ -1,7 +0,0 @@
|
|||
/**
|
||||
* Created by micku7zu on 1/27/2017.
|
||||
* Original idea: http://gijsroge.github.io/tilt.js/
|
||||
* MIT License.
|
||||
* Version 1.0.0
|
||||
*/
|
||||
"use strict";(function(){class b{constructor(c,d={}){if(!(c instanceof Node))throw"Can't initialize VanillaTilt because "+c+" is not a Node.";this.width=null,this.height=null,this.left=null,this.top=null,this.transitionTimeout=null,this.updateCall=null,this.updateBind=this.update.bind(this),this.element=c,this.settings=this.extendSettings(d),this.addEventListeners()}addEventListeners(){this.onMouseEnterBind=this.onMouseEnter.bind(this),this.onMouseMoveBind=this.onMouseMove.bind(this),this.onMouseLeaveBind=this.onMouseLeave.bind(this),this.element.addEventListener("mouseenter",this.onMouseEnterBind),this.element.addEventListener("mousemove",this.onMouseMoveBind),this.element.addEventListener("mouseleave",this.onMouseLeaveBind)}removeEventListeners(){this.element.removeEventListener("mouseenter",this.onMouseEnterBind),this.element.removeEventListener("mousemove",this.onMouseMoveBind),this.element.removeEventListener("mouseleave",this.onMouseLeaveBind)}destroy(){this.removeEventListeners(),this.element.vanillaTilt=null,this.element=null}onMouseEnter(){this.width=this.element.offsetWidth,this.height=this.element.offsetHeight,this.left=this.element.offsetLeft,this.top=this.element.offsetTop,this.element.style.willChange="transform",this.setTransition()}onMouseMove(c){null!==this.updateCall&&cancelAnimationFrame(this.updateCall),this.event=c,this.updateCall=requestAnimationFrame(this.updateBind)}onMouseLeave(){this.setTransition(),this.settings.reset&&this.reset()}reset(){requestAnimationFrame(()=>{this.event={pageX:this.left+this.width/2,pageY:this.top+this.height/2},this.element.style.transform="perspective("+this.settings.perspective+"px) rotateX(0deg) rotateY(0deg) scale3d(1, 1, 1)"})}getValues(){let c=(this.event.pageX-this.left)/this.width,d=(this.event.pageY-this.top)/this.height;c=Math.min(Math.max(c,0),1),d=Math.min(Math.max(d,0),1);let f=(this.settings.max/2-c*this.settings.max).toFixed(2),g=(d*this.settings.max-this.settings.max/2).toFixed(2);return{tiltX:f,tiltY:g,percentageX:100*c,percentageY:100*d}}update(){let c=this.getValues();this.element.style.transform="perspective("+this.settings.perspective+"px) rotateX("+("x"===this.settings.axis?0:c.tiltY)+"deg) rotateY("+("y"===this.settings.axis?0:c.tiltX)+"deg) scale3d("+this.settings.scale+", "+this.settings.scale+", "+this.settings.scale+")",this.element.dispatchEvent(new CustomEvent("tiltChange",{detail:c})),this.updateCall=null}setTransition(){clearTimeout(this.transitionTimeout),this.element.style.transition=this.settings.speed+"ms "+this.settings.easing,this.transitionTimeout=setTimeout(()=>this.element.style.transition="",this.settings.speed)}extendSettings(c){let d={max:20,perspective:1e3,easing:"cubic-bezier(.03,.98,.52,.99)",scale:"1",speed:"300",transition:!0,axis:null,reset:!0},f={};for(var g in d)if(g in c)f[g]=c[g];else if(this.element.hasAttribute("data-tilt-"+g)){let h=this.element.getAttribute("data-tilt-"+g);try{f[g]=JSON.parse(h)}catch(i){f[g]=h}}else f[g]=d[g];return f}static init(c,d){c instanceof Node&&(c=[c]),c instanceof NodeList&&(c=[].slice.call(c));c instanceof Array&&c.forEach(f=>{f.vanillaTilt=new b(f,d)})}}window.VanillaTilt=b,b.init(document.querySelectorAll("[data-tilt]"))})();
|
18
index.html
18
index.html
|
@ -3,11 +3,11 @@
|
|||
<html lang="en">
|
||||
<head>
|
||||
<meta charset="UTF-8">
|
||||
<title>vanillaTilt.js</title>
|
||||
<title>vanilla-tilt.js</title>
|
||||
<link href="https://fonts.googleapis.com/css?family=Source+Sans+Pro:300,400,400i,600,700" rel="stylesheet">
|
||||
<link href="https://fonts.googleapis.com/css?family=Fira+Sans+Extra+Condensed" rel="stylesheet">
|
||||
<meta name="description"
|
||||
content="vanillaTilt.js, a small easy to use 60+fps requestAnimationFrame powered parallax tilt effect without any dependencies inspired by Tilt.js. ">
|
||||
content="vanilla-tilt.js, a small easy to use 60+fps requestAnimationFrame powered parallax tilt effect without any dependencies inspired by Tilt.js. ">
|
||||
|
||||
<style>
|
||||
@charset "UTF-8";
|
||||
|
@ -2862,10 +2862,10 @@
|
|||
<body class="u-flex-set">
|
||||
|
||||
<aside>
|
||||
<a href="https://github.com/micku7zu/vanillaTilt.js" class="c-logo js-tilt" data-tilt-speed="400" data-tilt-scale="1.1" data-tilt-maxtilt="50"
|
||||
<a href="https://github.com/micku7zu/vanilla-tilt.js" class="c-logo js-tilt" data-tilt-speed="400" data-tilt-scale="1.1" data-tilt-maxtilt="50"
|
||||
data-tilt-perspective="500">
|
||||
<span class="backdrop"></span>
|
||||
<span>vanillaTilt.js</span>
|
||||
<span>vanilla-tilt.js</span>
|
||||
</a>
|
||||
</aside>
|
||||
<main>
|
||||
|
@ -2874,7 +2874,7 @@
|
|||
All credits goes to <strong><a href="http://gijsroge.github.io/">Gijs Rogé</a></strong>, he made the Tilt.js library based on jQuery.
|
||||
</p>
|
||||
<p class="u-font-huge">
|
||||
vanillaTils.js is Tilt.js but without jQuery dependency. Everything else is copied from Tilt.js!
|
||||
vanilla-tilt.js is Tilt.js but without jQuery dependency. Everything else is copied from Tilt.js!
|
||||
</p>
|
||||
<p class="u-font-large">
|
||||
Inspired, copied, forked, rewritten from <a href="http://gijsroge.github.io/tilt.js/">http://gijsroge.github.io/tilt.js/</a>
|
||||
|
@ -2885,8 +2885,8 @@
|
|||
<ul>
|
||||
<li>No Javascript dependencies</li>
|
||||
<li>No CSS is needed</li>
|
||||
<li><a href="https://github.com/micku7zu/vanillaTilt.js"
|
||||
title="Extra documentation about vanillaTilt.js plugin">Documentation</a></li>
|
||||
<li><a href="https://github.com/micku7zu/vanilla-tilt.js"
|
||||
title="Extra documentation about vanilla-tilt.js plugin">Documentation</a></li>
|
||||
</ul>
|
||||
|
||||
</header>
|
||||
|
@ -2899,7 +2899,7 @@
|
|||
<div class="c-example__info">
|
||||
<h3>The most basic usage:</h3>
|
||||
<ol>
|
||||
<li>Add <code class="language-html"><script src="vanillaTilt.js"></script></code>
|
||||
<li>Add <code class="language-html"><script src="vanilla-tilt.js"></script></code>
|
||||
right before the closing <code class="language-html"></body></code> tag
|
||||
</li>
|
||||
<li>Mark your elements with <code class="language-html"><span data-tilt></span></code>
|
||||
|
@ -3021,7 +3021,7 @@ element.addEventListener("tiltChange", function(event){ console.log(event.detail
|
|||
</article>
|
||||
|
||||
</main>
|
||||
<script src="dest/vanillaTilt.js"></script>
|
||||
<script src="src/vanilla-tilt.js"></script>
|
||||
<script>
|
||||
var elements = document.querySelectorAll(".js-tilt");
|
||||
var tiltOutput = document.querySelector(".js-tilt-output");
|
||||
|
|
Loading…
Reference in a new issue